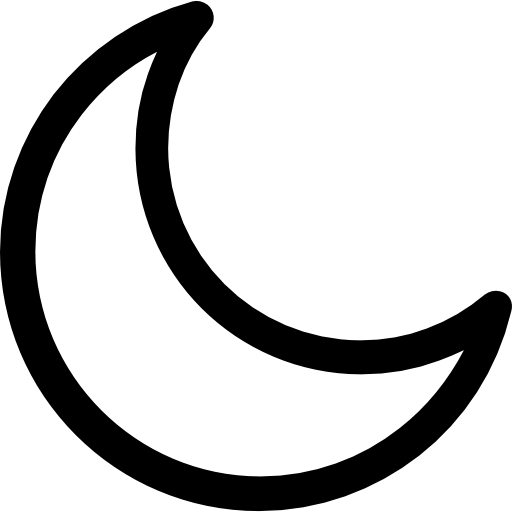
How to make a Kakoune plugin
Recently, I have fallen in love with the text editor Kakoune. The shortcuts make sense to me and I enjoy modal editing (switching between insert, normal, visual modes). But, NeoVim has an Obsidian plugin that I really enjoy, which Kakoune does not have.
So, I took matters into my own hands and made my own. I hope in this article I can teach you how to make your own very simple plugin.
Setup
Go to the .config/kak/plugins/ folder and create a directory for your plugin. In it, create a file with the .kak extention, this will be where the code of your plugin will be stored. In your kakrc include the following code:
source ~.config/kak/plugins/your-dir-name/your-plugin-name.kak do sh%{}
This allows Kakoune to implement the changes your plugin makes. Whenever you want your updates to be applied exit and enter back into Kakoune.
Making your first command
We will be making a simple "Hello World" plugin, where the user enters the command: :hello-world and "Hello World" will appear at the bottom of the screen. In the .kak file, the first line should be:
declare-user-mode your-file-name
User Mode allows the user to store custom mappings with no risk to shadow builtin ones. This is perfect for making commands for a plugin. Using define-command we can create a new command (as the name suggests). We can add the -docstring switch to show the documentation string for the command.
define-command -docstring "Prints 'Hello World'" hello-world %{}
To make the command actually usable, add the code to your file below:
map global user n :hello-world
The whole file should look like this:
declare-user-mode your-file-name
define-command -docstring "Prints 'Hello World'" hello-world %{}
map global user n :hello-world
If you restart Kakoune, you should be able to use the :hello-world command, but it doesn't do anything. Thankfully, we can use bash in Kakoune scripts using evaluate-commands as shown below:
define-command -docstring "Prints 'Hello World'" hello-world %{
evaluate-commands %sh{
}
}
In the %sh{} bash commands can be put in. To print "Hello World" at the bottom of the screen we can use echo:
define-command -docstring "Prints 'Hello World'" hello-world %{
evaluate-commands %sh{
echo 'echo -markup Hello World'
}
}
The echo command is used to enter Kakoune commands. The command to be entered, echo -markup Hello World, allows for Hello World to be printed at the bottom of the screen. The -markup switch allows for display text in an information box. The full code should be as follows:
declare-user-mode your-file-name
define-command -docstring "Prints 'Hello World'" hello-world %{
evaluate-commands %sh{
echo 'echo -markup Hello World'
}
}
map global user n :hello-world
If Kakoune is restarted and the :hello-world command is entered "Hello World" should be shown at the bottom of the screen. We have made our first command.
Wrapping Up
In this article we made a very simple plugin with a simple Hello World command. Hopefully the reader will use this information to make their own plugins. For more info check out the documentation in Kakoune's github repo in kakoune/doc/pages/commands.asciidoc.